children
Get the children of each DOM element within a set of DOM elements.
The querying behavior of this command matches exactly how
.children()
works in jQuery.
Syntax
.children()
.children(selector)
.children(options)
.children(selector, options)
Usage
Correct Usage
cy.get('nav').children() // Yield children of nav
Incorrect Usage
cy.children() // Errors, cannot be chained off 'cy'
cy.clock().children() // Errors, 'clock' does not yield DOM elements
Arguments
selector (String selector)
A selector used to filter matching DOM elements.
options (Object)
Pass in an options object to change the default behavior of .children()
.
Option | Default | Description |
---|---|---|
log | true | Displays the command in the Command log |
timeout | defaultCommandTimeout | Time to wait for .children() to resolve before timing out |
Yields
-
.children()
yields the new DOM element(s) it found.
Examples
No Args
.secondary-nav
Get the children of the <ul>
<li>About</li>
<li>
Services
<ul class="secondary-nav">
<li class="services-1">Web Design</li>
<li class="services-2">Logo Design</li>
<li class="services-3">
Print Design
<ul class="tertiary-nav">
<li>Signage</li>
<li>T-Shirt</li>
<li>Business Cards</li>
</ul>
</li>
</ul>
</li>
<li>Contact</li>
</ul>
// yields [
// <li class="services-1">Web Design</li>,
// <li class="services-2">Logo Design</li>,
// <li class="services-3">Print Design</li>
// ]
cy.get('ul.secondary-nav').children()
Selector
active
Get the children with class <div>
<ul>
<li class="active">Unit Testing</li>
<li>Integration Testing</li>
</ul>
</div>
// yields [
// <li class="active">Unit Testing</li>
// ]
cy.get('ul').children('.active')
Rules
Requirements
-
.children()
requires being chained off a command that yields DOM element(s).
Assertions
-
.children()
will automatically retry until the element(s) exist in the DOM -
.children()
will automatically retry until all chained assertions have passed
Timeouts
-
.children()
can time out waiting for the element(s) to exist in the DOM . -
.children()
can time out waiting for assertions you've added to pass.
Command Log
Assert that there should be 8 children elements in a nav
cy.get('.left-nav>.nav').children().should('have.length', 8)
The commands above will display in the Command Log as:

When clicking on the children
command within the command log, the console
outputs the following:
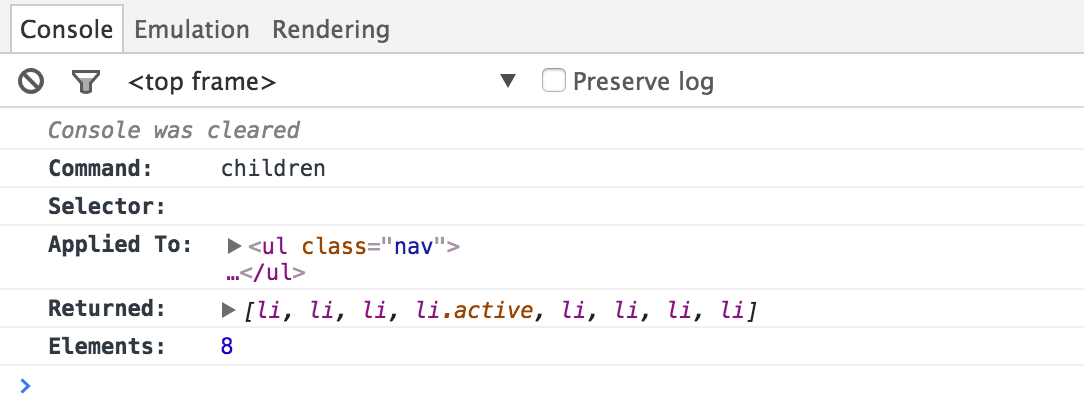