filter
Get the DOM elements that match a specific selector.
Opposite of .not()
The querying behavior of this command matches exactly how
.filter()
works in jQuery.
Syntax
.filter(selector)
.filter(selector, options)
Usage
Correct Usage
cy.get('td').filter('.users') // Yield all el's with class '.users'
Incorrect Usage
cy.filter('.animated') // Errors, cannot be chained off 'cy'
cy.clock().filter() // Errors, 'clock' does not yield DOM elements
Arguments
selector (String selector)
A selector used to filter matching DOM elements.
options (Object)
Pass in an options object to change the default behavior of .filter()
.
Option | Default | Description |
---|---|---|
log | true | Displays the command in the Command log |
timeout | defaultCommandTimeout | Time to wait for .filter() to resolve before timing out |
Yields
-
.filter()
yields the new DOM element(s) it found.
Examples
Selector
Filter the current subject to the elements with the class 'active'
<ul>
<li>Home</li>
<li class="active">About</li>
<li>Services</li>
<li>Pricing</li>
<li>Contact</li>
</ul>
// yields <li>About</li>
cy.get('ul').find('>li').filter('.active')
Contains
Filter by text
You can use the jQuery
selector to perform a case-sensitive text substring match.<ul>
<li>Home</li>
<li>Services</li>
<li>Advanced Services</li>
<li>Pricing</li>
<li>Contact</li>
</ul>
Let's find both list items that contain the work "Services"
cy.get('li').filter(':contains("Services")').should('have.length', 2)
Non-breaking space
If the HTML contains a
non-breaking space entity
&nbsp;
and the test uses the
jQuery
\u00a0
instead of &nbsp;
.
<div data-testid="testattr">
<span>Hello&nbsp;world</span>
</div>
cy.get('[data-testid="testattr"]').filter(':contains("Hello\u00a0world")')
Rules
Requirements
-
.filter()
requires being chained off a command that yields DOM element(s).
Assertions
-
.filter()
will automatically retry until the element(s) exist in the DOM -
.filter()
will automatically retry until all chained assertions have passed
Timeouts
-
.filter()
can time out waiting for the element(s) to exist in the DOM . -
.filter()
can time out waiting for assertions you've added to pass.
Command Log
Filter the li's to the li with the class 'active'.
cy.get('.left-nav>.nav').find('>li').filter('.active')
The commands above will display in the Command Log as:

When clicking on the filter
command within the command log, the console
outputs the following:
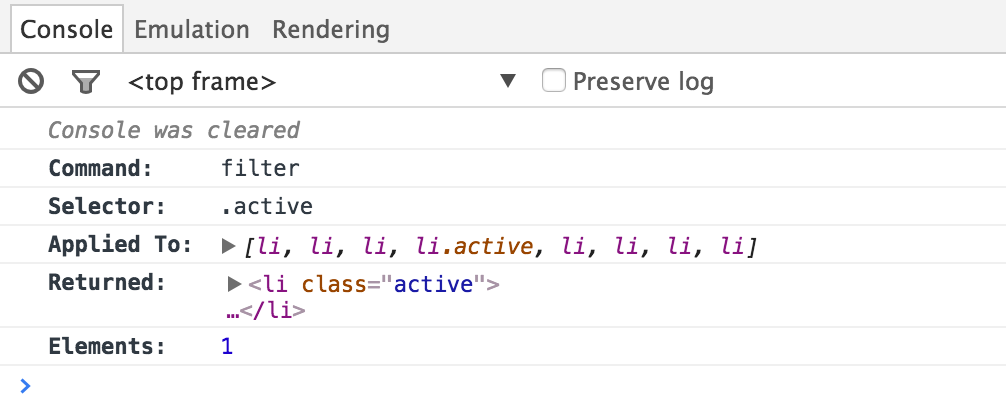