clock
cy.clock()
overrides native global functions related to time allowing them to
be controlled synchronously via cy.tick()
or the yielded
clock
object. This includes controlling:
setTimeout
clearTimeout
setInterval
clearInterval
Date
Objects
The clock starts at the unix epoch (timestamp of 0). This means that when you
instantiate new Date
in your application, it will have a time of
January 1st, 1970
.
Syntax
cy.clock()
cy.clock(now)
cy.clock(now, functionNames)
cy.clock(options)
cy.clock(now, options)
cy.clock(now, functionNames, options)
Usage
Correct Usage
cy.clock()
Arguments
now (number)
A timestamp specifying where the clock should start.
functionNames (Array)
Name of native functions that clock should override.
options (Object)
Pass in an options object to change the default behavior of cy.clock()
.
Option | Default | Description |
---|---|---|
log | true | Displays the command in the Command log |
Yields
cy.clock()
yields a clock
object with the following methods:
-
clock.tick(milliseconds)
Move the clock the specified number of
milliseconds
. Any timers within the affected range of time will be called. -
clock.restore()
Restore all overridden native functions. This is automatically called between tests, so should not generally be needed.
-
clock.setSystemTime(now)
Change the system time to the new
now
. Now can be a timestamp, date object, or not passed in which defaults to 0. No timers will be called, nor will the time left before they trigger change.
You can also access the clock
object via this.clock
in a
.then()
callback.
Examples
No Args
setInterval
Create a clock and use it to trigger a // your app code
let seconds = 0
setInterval(() => {
$('#seconds-elapsed').text(++seconds + ' seconds')
}, 1000)
cy.clock()
cy.visit('/index.html')
cy.tick(1000)
cy.get('#seconds-elapsed').should('have.text', '1 seconds')
cy.tick(1000)
cy.get('#seconds-elapsed').should('have.text', '2 seconds')
Access the clock object to synchronously move time
In most cases, it's easier to use cy.tick()
to move
time, but you can also use the clock
object yielded by cy.clock()
.
cy.clock().then((clock) => {
clock.tick(1000)
})
You can call cy.clock()
again for this purpose later in a chain if necessary.
cy.clock()
cy.get('input').type('Jane Lane')
cy.clock().then((clock) => {
clock.tick(1000)
})
The clock object is also available via this.clock
in any
.then()
callback.
cy.clock()
cy.get('form').then(($form) => {
this.clock.tick(1000)
// do something with $form ...
})
Access the clock object to restore native functions
In general, it should not be necessary to manually restore the native functions
that cy.clock()
overrides since this is done automatically between tests. But
if you need to, the clock
object yield has a .restore()
method.
cy.clock().then((clock) => {
clock.restore()
})
Or via this.clock
:
cy.clock()
cy.get('.timer').then(($timer) => {
this.clock.restore()
// do something with $timer ...
})
Now
Specify a now timestamp
const now = new Date(2021, 3, 14) // month is 0-indexed
cy.clock(now)
cy.visit('/index.html')
cy.get('#date').should('have.value', '04/14/2021')
const now = new Date(2021, 3, 14) // month is 0-indexed
cy.clock(now)
cy.mount(<DatePicker id="date" />)
cy.get('#date').should('have.value', '04/14/2021')
Function names
Specify which functions to override
This example below will only override setTimeout
and clearTimeout
and leave
the other time-related functions as they are.
cy.clock(null, ['setTimeout', 'clearTimeout'])
Note that you must specify Date
in order to override the current datetime. The
example below affects the current datetime without affecting scheduled timers.
cy.clock(Date.UTC(2018, 10, 30), ['Date'])
Using cy.clock()
with cy.tick()
Restore clock
You can restore the clock and allow your application to resume normally without manipulating native global functions related to time. This is automatically called between tests.
cy.clock()
cy.visit('http://localhost:3333')
cy.get('#search').type('Acme Company')
cy.tick(1000)
// more test code here
// restore the clock
cy.clock().then((clock) => {
clock.restore()
})
// more test code here
You could also restore by using .invoke() to invoke the
restore
function.
cy.clock().invoke('restore')
Change current system time
Here we test that a timer still looks good if it has run for an hour, without triggering an hours worth of setInterval or requestAnimationFrame timers and overloading our CPU.
cy.clock(0)
cy.visit('http://localhost:3333')
cy.clock().then((clock) => {
clock.setSystemTime(60 * 60 * 1000 - 60);
// setSystemTime doesn't trigger any timers, so we run the last frame
// with tick to trigger a callback to update the timer.
clock.tick(60);
})
cy.get('#timer').should(...) // assert that it fits within the screen etc.
// more test code here
You could also change the system time by using .invoke()
to invoke the setSystemTime
function.
cy.clock().invoke('setSystemTime', 60 * 60 * 1000)
Notes
iframes
iframes not supported
Note that cy.clock()
only applies to the top
window on a web page. It will
not override the time functions of any iframe
embedded on the page.
Behavior
cy.mount()
clock behavior before Using the cy.mount()
command in a Cypress Component
Test will render your component but does not affect the behavior of the page or
window object. This means you can mount
directly after calling cy.clock()
to
test the component against any changes you've made to the yielded clock object.
cy.visit()
clock behavior before If you call cy.clock()
before visiting a page with
cy.visit()
, the page's native global functions will be
overridden on window load, before any of your app code runs. So even if
setTimeout
, for example, is called on page load, it can still be controlled
via cy.tick()
. This also applies if, during the course
of a test, the page under test is reloaded or changed.
Rules
Requirements
-
cy.clock()
requires being chained off ofcy
.
Assertions
-
cy.clock()
is a utility command. -
cy.clock()
will not run assertions. Assertions will pass through as if this command did not exist.
Timeouts
-
cy.clock()
cannot time out.
Command Log
Create a clock and tick it 1 second
cy.clock()
cy.tick(1000)
The command above will display in the Command Log as:
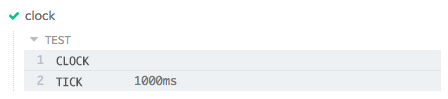
When clicking on the clock
command within the command log, the console outputs
the following:

History
Version | Changes |
---|---|
10.7.0 | Added setSystemTime to yielded clock object |