spy
Wrap a method in a spy in order to record calls to and arguments of the function.
Note: .spy()
assumes you are already familiar with our guide:
Stubs, Spies, and Clocks
Syntax
cy.spy(object, method)
Usage
Correct Usage
cy.spy(user, 'addFriend')
Arguments
object (Object)
The object
that has the method
to be wrapped.
method (String)
The name of the method
on the object
to be wrapped.
Yields
Unlike most Cypress commands, cy.spy()
is synchronous and returns a value
(the spy) instead of a Promise-like chain-able object.
cy.spy()
returns a Sinon.js spy.
All methods found on Sinon.JS spies are supported.
Examples
Method
Wrap a method with a spy
// assume App.start calls util.addListeners
cy.spy(util, 'addListeners')
App.start()
expect(util.addListeners).to.be.called
Disable logging to Command Log
You can chain a .log(bool)
method to disable cy.stub()
calls from being
shown in the Command Log. This may be useful when your stubs are called an
excessive number of times.
const obj = {
foo() {},
}
const stub = cy.stub(obj, 'foo').log(false)
cy.spy()
examples
More
Aliases
Adding an alias using .as()
to spies makes them easier to
identify in error messages and Cypress' command log.
const obj = {
foo() {},
}
const spy = cy.spy(obj, 'foo').as('anyArgs')
const withFoo = spy.withArgs('foo').as('withFoo')
obj.foo()
expect(spy).to.be.called
expect(withFoo).to.be.called // purposefully failing assertion
You will see the following in the command log:
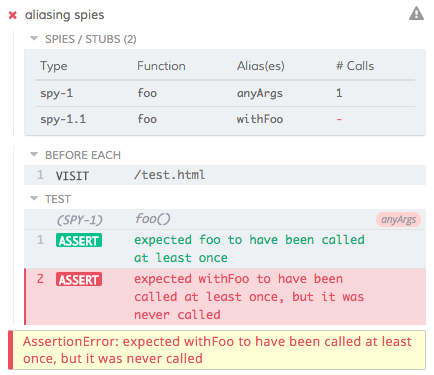
Notes
Restores
Automatic reset/restore between tests
cy.spy()
creates spies in a
sandbox, so all spies created
are automatically reset/restored between tests without you having to explicitly
reset/restore them.
Differences
Difference between cy.spy() and cy.stub()
The main difference between cy.spy()
and cy.stub()
is
that cy.spy()
does not replace the method, it only wraps it. So, while
invocations are recorded, the original method is still called. This can be very
useful when testing methods on native browser objects. You can verify a method
is being called by your test and still have the original method action invoked.
Assertions
Assertion Support
Cypress has also built-in
Sinon-Chai support, so any
assertions supported by Sinon-Chai
can be used without any configuration.
Rules
Requirements
-
cy.spy()
requires being chained off ofcy
.
Assertions
-
cy.spy()
cannot have any assertions chained.
Timeouts
-
cy.spy()
cannot time out.
Command Log
Create a spy, alias it, and call it
const obj = {
foo() {},
}
const spy = cy.spy(obj, 'foo').as('foo')
obj.foo('foo', 'bar')
expect(spy).to.be.called
The command above will display in the Command Log as:
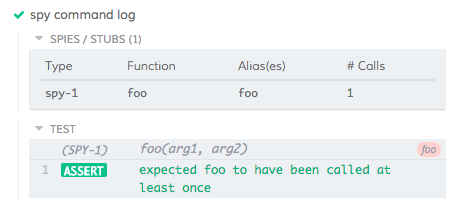
When clicking on the spy-1
event within the command log, the console outputs
the following:
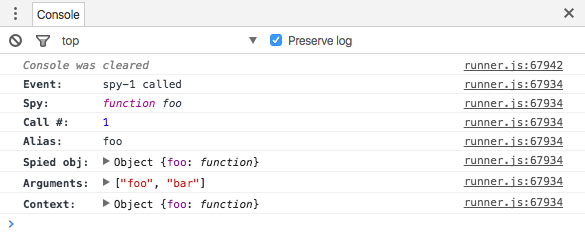
History
Version | Changes |
---|---|
0.20.0 | Added .log(bool) method |
0.18.8 | cy.spy() command added |